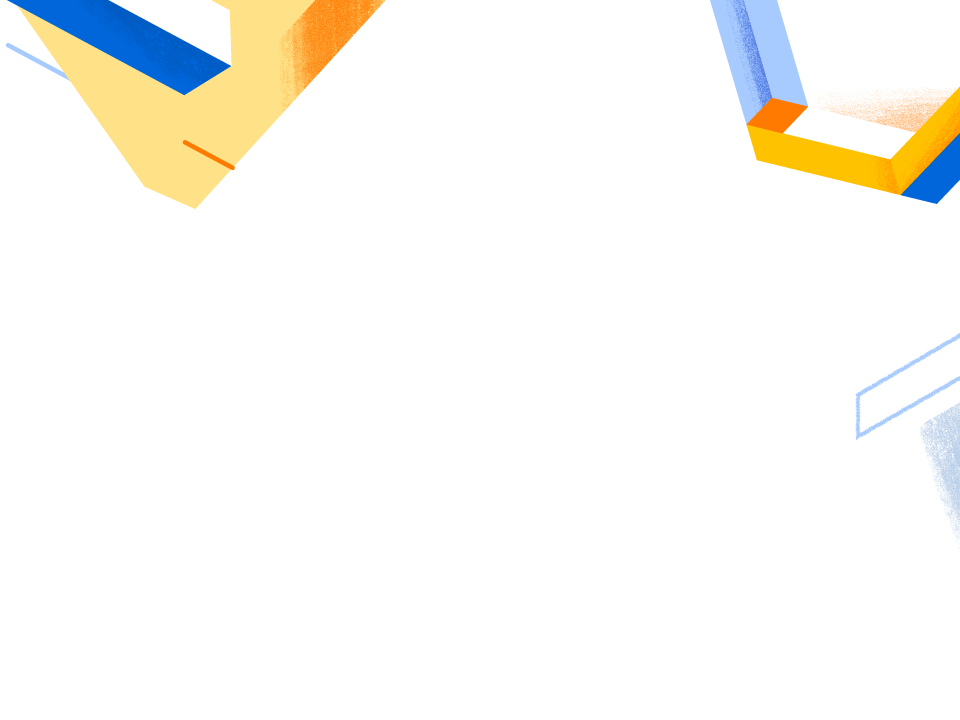
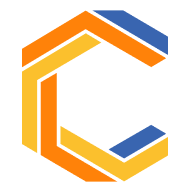
Cirq
An open source framework for programming quantum computers
Cirq is a Python software library for writing, manipulating, and optimizing quantum circuits, and then running them on quantum computers and quantum simulators. Cirq provides useful abstractions for dealing with today’s noisy intermediate-scale quantum computers, where details of the hardware are vital to achieving state-of-the-art results.
Get started with Cirq
GitHub repository
import cirq # Pick a qubit. qubit = cirq.GridQubit(0, 0) # Create a circuit circuit = cirq.Circuit( cirq.X(qubit)**0.5, # Square root of NOT. cirq.measure(qubit, key='m') # Measurement. ) print("Circuit:") print(circuit) # Simulate the circuit several times. simulator = cirq.Simulator() result = simulator.run(circuit, repetitions=20) print("Results:") print(result)
Features
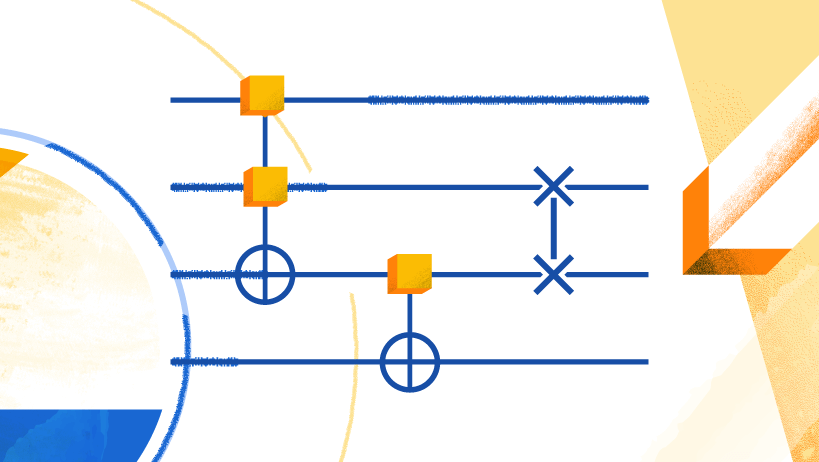
Circuits
Learn how to build quantum circuits from gates acting on qubits. Understand what a Moment is and how different insertion strategies can help you build your desired circuit. Learn about how to slice and dice circuits and transform them into new and better circuits.
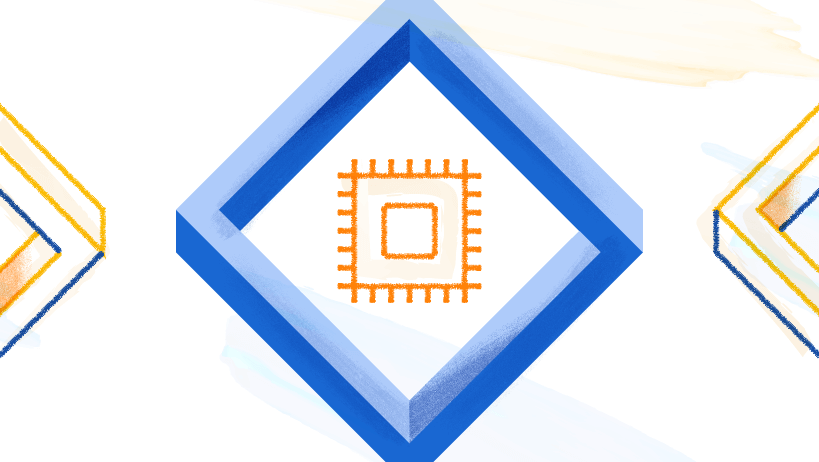
Devices
Hardware constraints have a large impact on whether a circuit is practical or not on modern hardware. Learn how devices can be defined to handle these constraints.
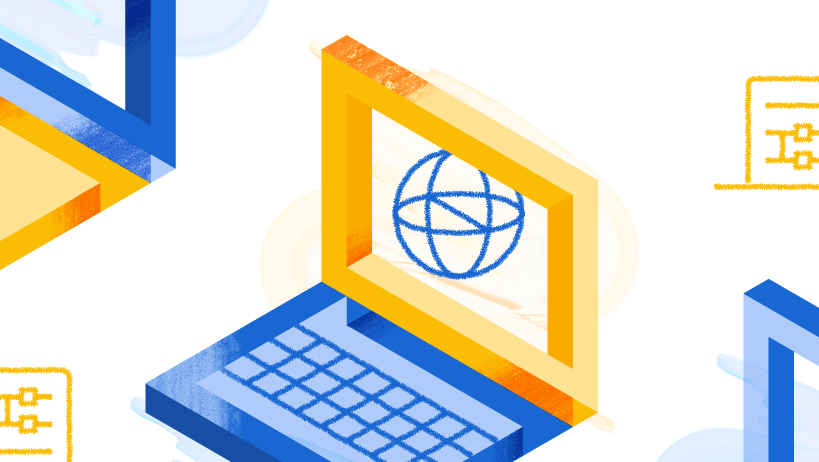
Simulation
Cirq comes with built-in simulators, both for wave functions and for density matrices. These can handle noisy quantum channels using monte carlo or full density matrix simulations. In addition, Cirq works with a state-of-the-art wave function simulator: qsim. These simulators can be used to mock quantum hardware with the Quantum Virtual Machine.
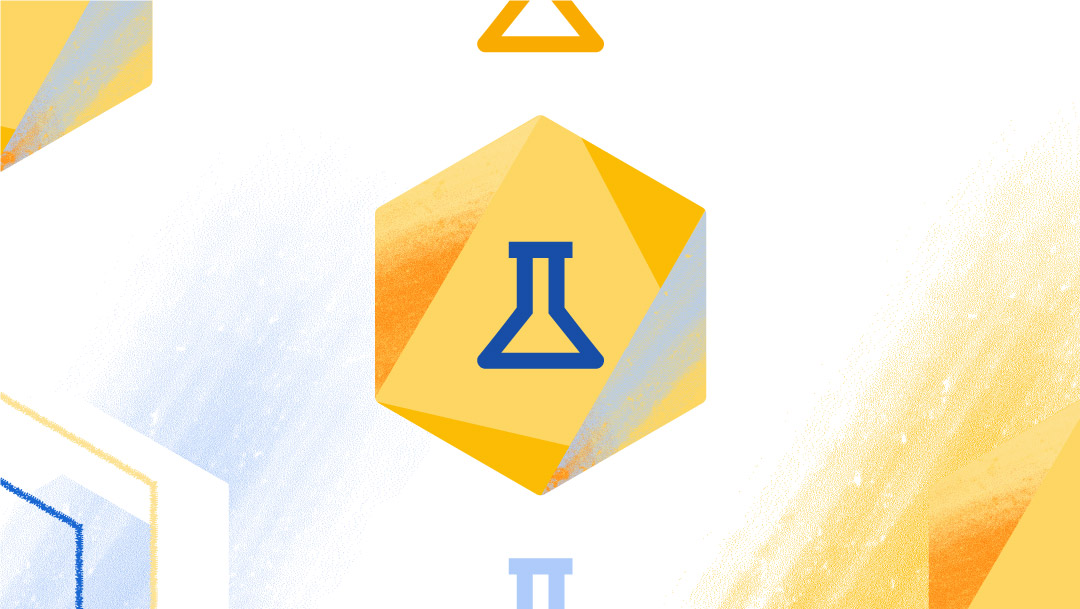
End-2-end experiments
Cirq is used to run experiments on Google's quantum processors. Learn more about the latest experiments and access the code to see how to run them yourself.
For beginners:
For advanced users:
Featured guides and tutorials
Introduction to Cirq
Learn the basics of Cirq, from gates to operations to circuits to simulation. This is the best starting point for those who know the basics of quantum computing.
Textbook algorithms
Cirq users have coded up a zoo of textbook quantum algorithms. These give you an idea of good patterns to follow in your own code and are useful when you are learning these algorithms.
Getting started with the QVM
The Quantum Virtual Machine gives you the opportunity to run circuits on simulated hardware, which mocks the circuit constraints and noise behavior present in existing quantum hardware devices.
QAOA
See how to implement a Quantum Approximate Optimization Algorithm on real hardware.
Resources
Community
We are dedicated to cultivating an open and inclusive community to build software for near term quantum computers, and we welcome contributions from the community.
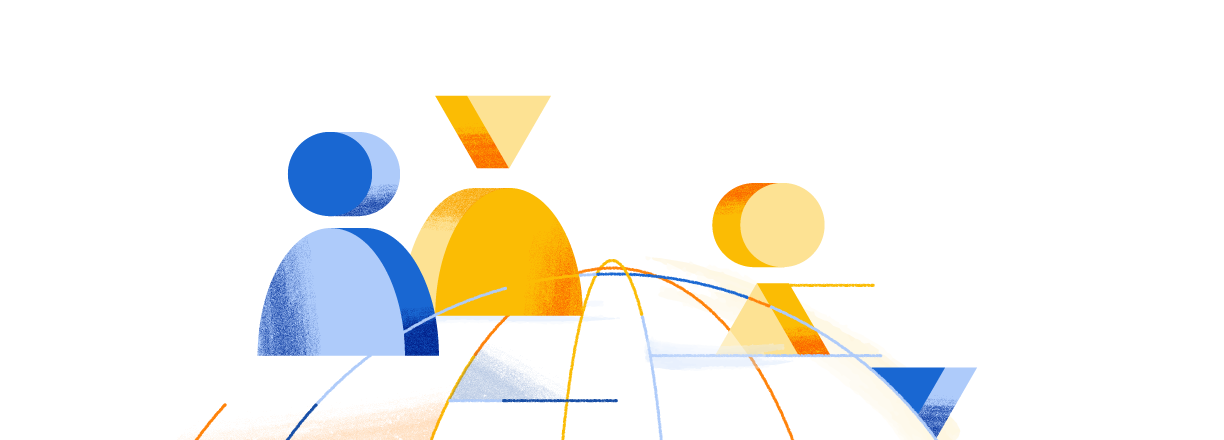
Cirq Weekly Sync
Subscribe: cirq-dev
OpenFermion Weekly Sync
Subscribe: openfermion-dev
TensorFlow Quantum Weekly Sync
Subscribe: tfq-dev
Quantum Circuit Simulation Weekly Sync
Subscribe: qsim-qsimh-dev
Request for comment
For larger features, check out our RFC process to learn how to contribute.